How to Make a Custom Hook in next js
rajneesh
5 min read
- react
- node js
- JavaScript
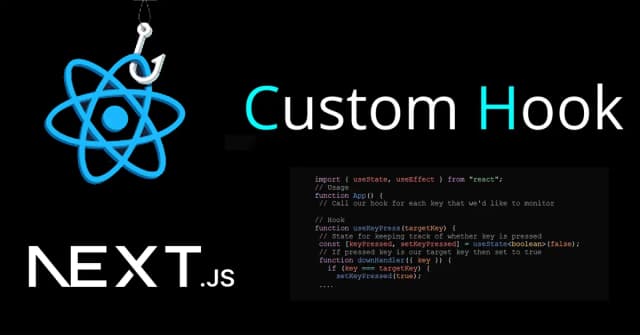
Something we need to share logic between different components without repeating code. At that time, we can create our own custom hook for that logic and use that hook with different components. Custom hooks allow you to extract and reuse logic across multiple components, making your code more modular and maintainable.
By doing this, you can easily reduce code repetition and achieve clear and easy-to-maintain code.
As a NextJS developer, you most know how to create custom hook,let start this post with a step-by-step guide.
What is a Custom Hook?
We know that hooks like useState and useEffect are reusable function components that can be used inside components. Sometimes we create components that we have to reuse again and again, just like that. We need to use some functional logic, then we can create hooks to reuse some functional logic.
Why Use Custom Hooks?
Code Reusability: Share logic between components without repeating code.
Separation of Concerns: Encapsulate related logic in a single function, making components cleaner and easier to maintain.
Improved Readability: Abstract complex logic into simpler functions.
Step-by-Step Guide to Creating a Custom Hook
In this guide, we are creating a custom hook for the CRUD operation on any API call. This hook provides you different functionalities like createData, getData,updateData and deleteData.
Setting Up Your React Project
Start with creating a next JS. You can use configuration according to your preference and adding reqired modules:
// create project command npx create-next-app@latest // next app configuration What is your project named? my-app Would you like to use TypeScript? No / Yes Would you like to use ESLint? No / Yes Would you like to use Tailwind CSS? No / Yes Would you like to use `src/` directory? No / Yes Would you like to use App Router? (recommended) No / Yes Would you like to customize the default import alias (@/*)? No / Yes What import alias would you like configured? @/* // change directory into project directory cd ./my-app //install module npm install axios
Creating Custom Hook
inside the
src
directory, create a news file /common/hook/api/Api.js like this :// src/common/hook/api/Api.js import { useState } from 'react'; import axios from 'axios'; import { useState } from 'react'; const useApi = (initialValue:object) => { const [data, setData] = useState(initialValue); const [loading, setLoading] = useState(false); const [error, setError] = useState<any>(); const getData = async (url:string) => { setLoading(true); try { const response = await axios(url); const data = response.data; setData(data); } catch (error) { console.error(error); setError(error); } finally { setLoading(false); } }; const postData = async (url:string, body:any) => { setLoading(true); try { const response = await axios.post(url, body); const data = response.data; setData(data); } catch (error) { console.error(error); setError(error); } finally { setLoading(false); } }; const putData = async (url:string, body:any) => { setLoading(true); try { const response = await axios.put(url, body); const data = response.data; setData(data); } catch (error) { console.error(error); setError(error); } finally { setLoading(false); } }; const deleteData = async (url:string) => { setLoading(true); try { const response = await axios.delete(url); const data = response.data; setData(data); } catch (error) { console.error(error); setError(error); } finally { setLoading(false); } }; return { data, loading, error , getData, postData, putData, deleteData }; }; export default useApi;
here we can create a custom hook useApi by which we can manages api data. The hook returns an object which is containing the curent api data if error is not accured, error, loading and some functions to getData,postData,putData,deleteData.
Using the Custom Hook in a Component
Now, let's use our custom hook in a React component. we are creating components file on file we create productSection components:
// src/common/components/ProductSection/ProductSection.js "use client" import React, { useEffect } from 'react' import useApi from '../../hooks/api/Api' import Card from '../Card/Card' const ProductSection = () => { const { data,loading,error,getData} = useApi({data:"",products:[{id:"",thumbnail:"",description:"",price:""}]}) useEffect(() => { getData("https://dummyjson.com/products?limit=12") }, []) console.log(data) return ( <div> <h2>Product Section</h2> {loading && <p>Loading...</p>} {error && <p>Error: {error.message}</p>} <div className='flex flex-wrap justify-center gap-4'> {data.products.length > 0 && data?.products.map((product:any) => ( <Card key={product.id} thumbnail={product.thumbnail} title={product.title} description={product.description} price={product.price} /> ))} </div> </div> ) } export default ProductSection
In
ProductSection component
, we import and use theuseApi
hook by which we can getting api data using getData function and also checking loading and error while api call. while loading the we are showing loading on screen if any error accor the we can show that error else data get successfuly the we are showing the data on screen with the help of card component.Integrating the Component
Finally, integrate the
productSection component
into your home page component:import ProductSection from "./common/components/ProductSecton/ProductSection"; export default function Home() { return ( <div> <ProductSection/> </div> ); }
now we can run the app and test the app using this commond:
npm run dev
Conclusion
custom hook is very useful while we need to use simple code multiple time then you must be create an custom hook for that. in this post i have create custom hook for handling the api crud operation like that you can also create hook for handling ecommerce cart data or managing count for verious place. by this you can also reuse any function in any other project by create that function into a any react hook. you can check my github account for complete code